π Object Finder
What is the Object Finder
- Find any specific GameObject in the hierarchy
- Get GameObjects from different prefabs in different scenes
Advantages of the Object Finder
- Subscribe to the creation of the object before it is created
- Completely decoupled way of getting GameObjects throughout the project
- Be able to assign any object to listen to, which reference could change over time, for example highlight any selected GameObject in the scene
- Be able to also Unregister an object
- Manually assign ids to spawned GameObjects
- Many systems use the Object Finder, such as the Sequence System, for example to track and follow objects which exist in a 3D scene on top of a 2D canvas, all without having direct references
How to Register an Object to the ObjectFinder
Add the RegisterObject Component to any GameObject and select an existing Object Id from the ObjectFinderLibrary dropdown.

If you need to register a new Object Id, simply switch to the Create New tab.
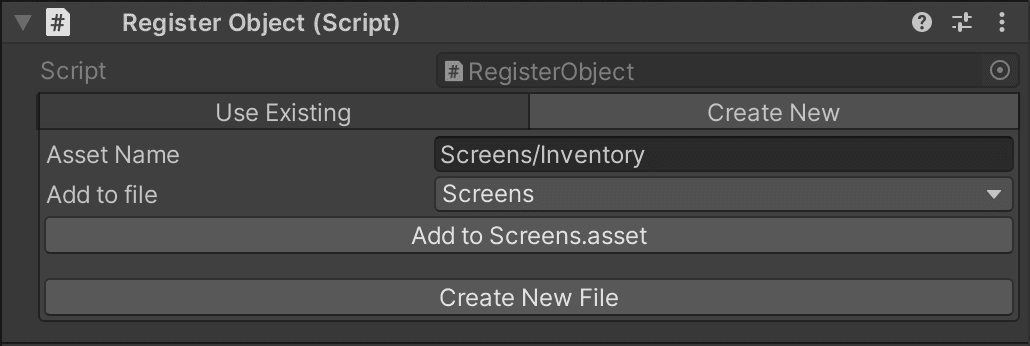
Asset Name
Write the name in the Asset Name field. Also include the sub folders for the dropdown in the name field, such as "Screens/Store/Daily Chest".
Add to existing file
Select to which existing ObjectFinderLibrary file you would like to append the new Object Id and press the Add to filename button.
Create new file
If you wish to create a new file, just enter the Asset Name and press Create New File to browse to a location where to store it in the project.
The new Object Id will get created and automatically assigned to this RegisterObject script, no further action required after creating a new Object Id.
How to access an Object
Access from code
Use these two methods to subscribe and unsubscribe, like in the example below:
ObjectFinderService.Subscribe(Guid objectId, Action<GameObject> callback)
ObjectFinderService.Unsubscribe(Guid objectId, Action<GameObject> callback)
using GameSuite;
namespace Game
{
public class HighlightObject : SerializedMonoBehaviour
{
[SerializeField, ObjectFinder]
private Guid objectId;
protected void Awake()
{
Subscribe();
}
protected void OnDestroy()
{
// Always Unsubscribe when Subscribing to anything.
Unsubscribe();
}
public void Subscribe()
{
if (objectId != Guid.Empty)
{
objectFinderService = Services.Get<ObjectFinderService>();
objectFinderService.Subscribe(objectId, ObjectFound);
}
}
public void Unsubscribe()
{
if (objectFinderService != null)
{
objectFinderService.Unsubscribe(objectId, ObjectFound);
objectFinderService = null;
}
}
private void ObjectFound(GameObject target)
{
// Do something with the target object.
// Cache the previous object, to hide the highlight on the previous object.
}
}
}
FindObject Component
Add the FindObject component to trigger UnityActions when it is found, passing in the GameObject as a parameter.
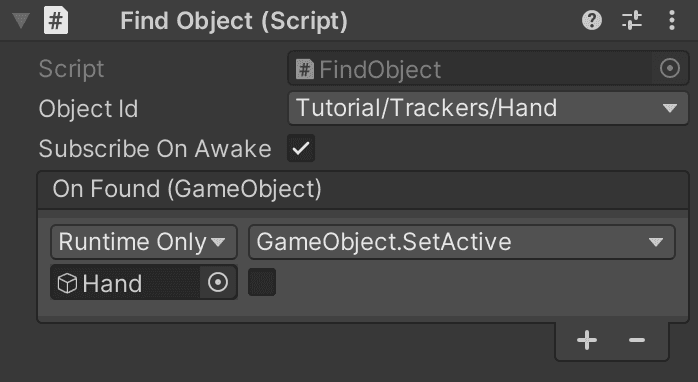
Variables and methods:
ObjectId
: Assign the Object Id from the dropdown you want to listen to.SubscribeOnAwake
: Listen for the object creation immediately or start listening manually when you decide it should start listening for the object being created.OnFound
: This Unity Event passes the GameObject to whatever script you like, or any action which needs to be executed.Subscribe()
: Manually subscribe waiting for the object creation.Unsubscribe()
: Manually stop listening for the object being created.
FindObject Flow Condition
In the Flow, you can wait for an object to be created and then proceed along a path in the flow. This example waits for the UI Camera to exist before proceeding to the Show UI Node:
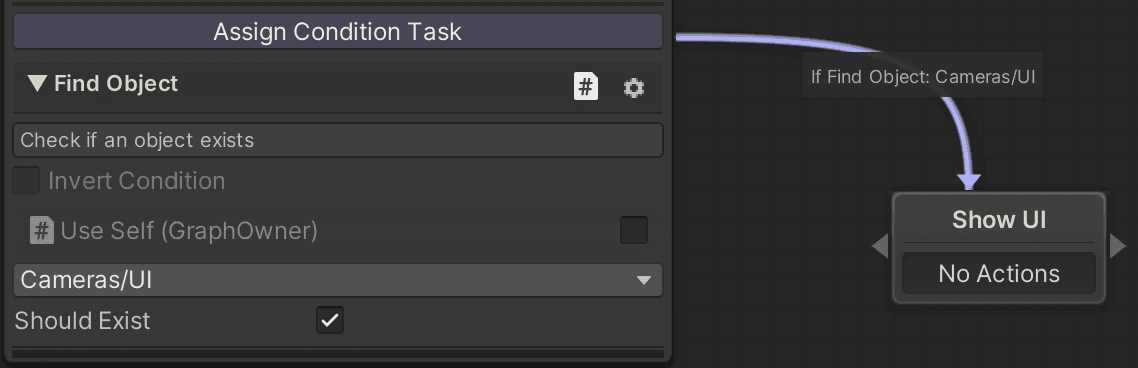
Uncheck the Should Exist box to wait for the object to be destroyed/unregistered, which is a reverse of waiting for it to exist.
Register from code
Registering an object from code is useful when for example instantiating lots of GameObjects, and one of them should be highlighted or picked.
[ObjectFinder]
public Guid ObjectId;
private RegisterObject registerObject;
protected void Start()
{
AddHighlight(gameObject);
}
public void AddHighlight(GameObject highlightObject)
{
registerObject = highlightObject.AddComponent<RegisterObject>();
registerObject.Register(ObjectId);
}
public void RemoveHighlight()
{
registerObject.Unregister();
}