Flow Conditions
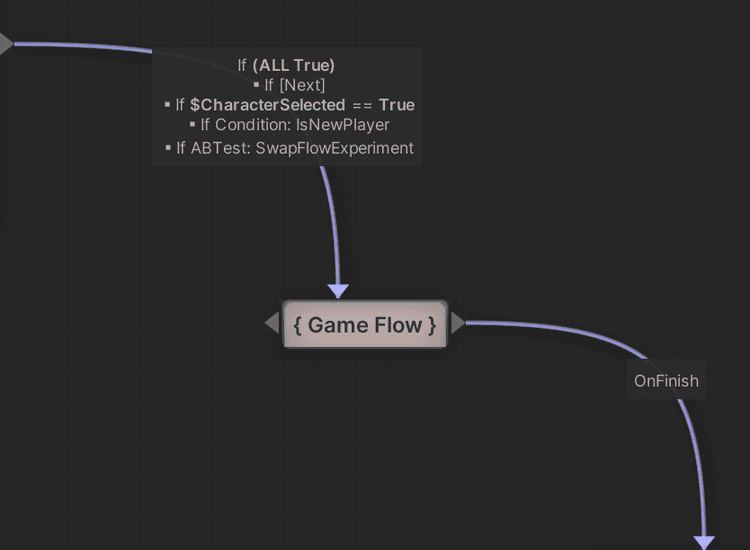
Flow conditions are small independent scripts that return a true or false.
These conditions can be assigned to any connection between nodes, which allows to check for number of different conditions that need to be valid to take a certain path to the next node.
Conditions can be stacked to support complex behaviors trees.
Disable transition
Each connection can be individually disabled. This is useful for features that are in development and do not want accessible yet. Press the Active
checkbox in the top left of the connection inspector.

Requirement
This is a setting which can be set for every individual connection. Either check if all conditions need to be true or a single one suffices to take this transition.
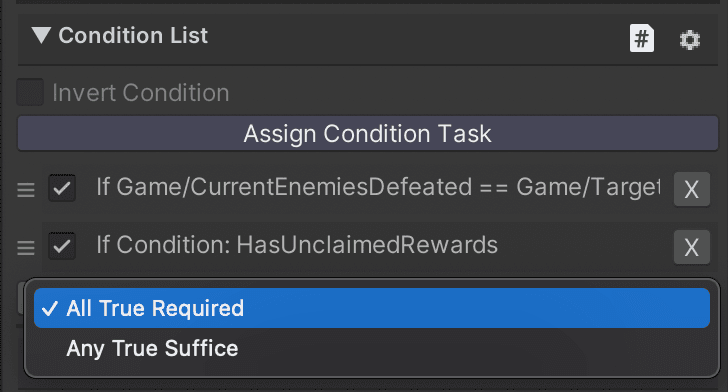
Invert Condition
Invert the entire Condition List or each individual Condition. Underneath the Condition List
and just under the Condition
inspector, by selecting a specific flow condition.
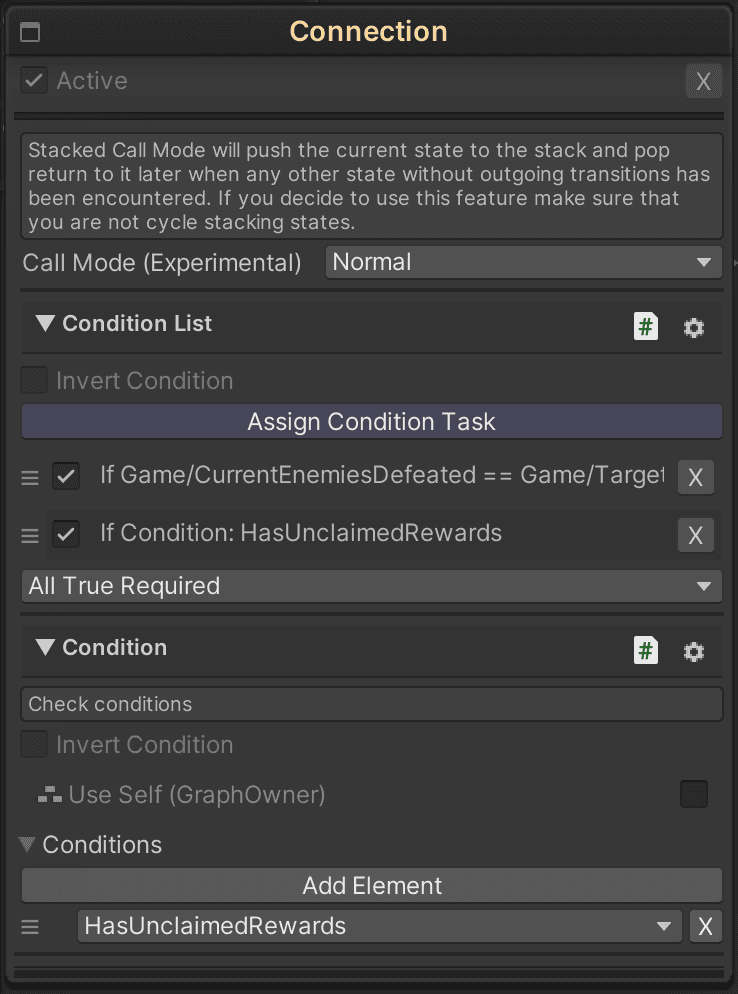
Rewiring
Either drag before the middle or after the middle of the connection line to rewire the beginning or the end of the connection.
OnFinish
By default, there are no conditions added to a node connection, in which case the OnFinish text appears on the transition. This implies that when the previous node is finished/green, this transition will be valid and jump onto the next node.
This is convenient when having to load a scene in one node, and when finished loading, the game should start.
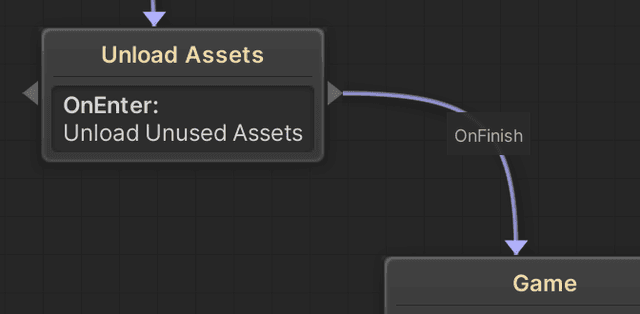
Flow Event
This is the most used event in the Flow Editor. It listens for Flow Events getting fired from prefabs, scenes, (flow) actions, debug menu or custom code.
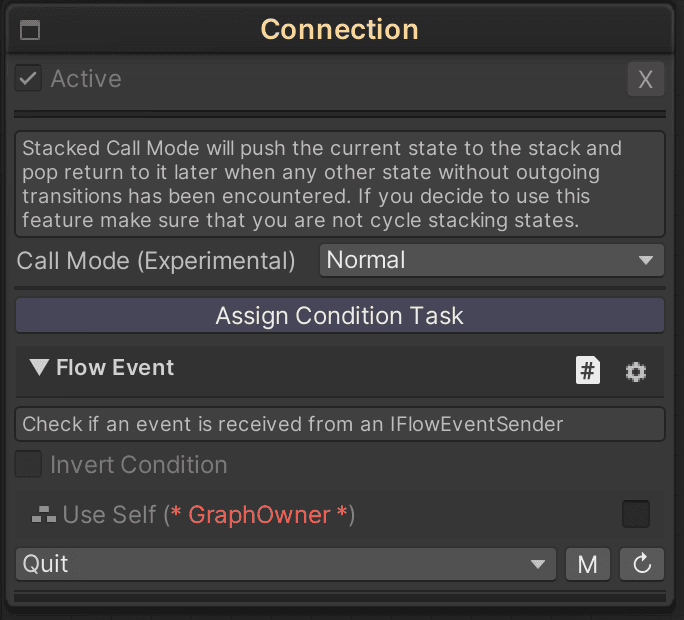
When adding a flow event from a node that spawns objects. The flow event dropdown menu will automatically get populated with the available events.
In case the flow event is coming from a different place in the application, you can enter a manual name by toggling the [M]
button in the inspector and writing the custom flow event name in the text box.
Services
Service Initialized
Check if a single or multiple services have been initialized before continuing the flow.
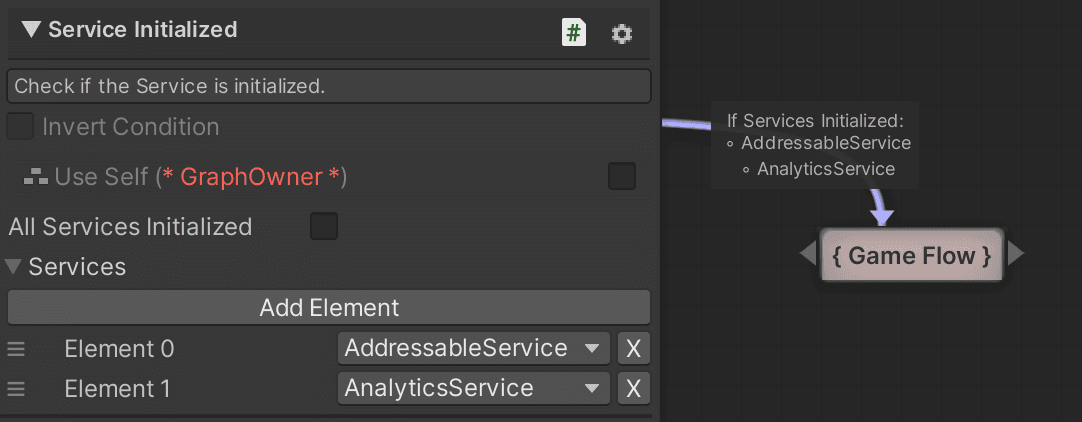
Use the checkbox on the inspector to check if all the services are initialized that implement IInitializable.
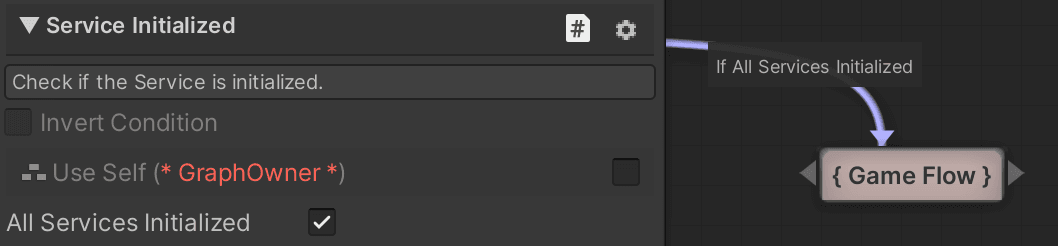
AB Test
Check if an experiment is active and choose a different path in the flow.
Check Internet Connection
Check if the user has an open internet connection.
Library Condition
Checks if a ConditionLibrary asset is valid, view the ConditionalAction chapter for more info.
Find Object
Tries to find an object through the ObjectFinder, when it can be located, the condition will be true.
Sequence Completed
Check if a sequence or a specific step within a sequence has been completed by the user.
Addressables
Addressables Caching Completed
Select which labels need to be cached in memory through a flow action and wait for it to complete using this flow condition.
Addressables Content Downloaded
All content requested through the flow action or code has been completed.
Whiteboard
Check if whiteboard variables are set, or set to a specific value. There are multiple build-in options but can be extended if required.
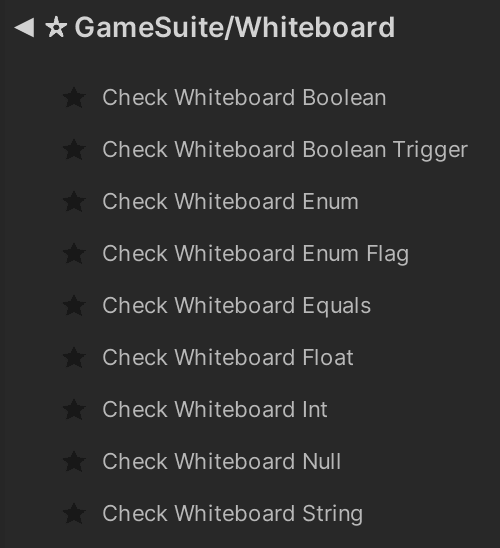
An example use case scenario would be checking if there are rewards to collect by checking if the RewardAmount
whiteboard value is greater than 0 and taking a different path in the flow:
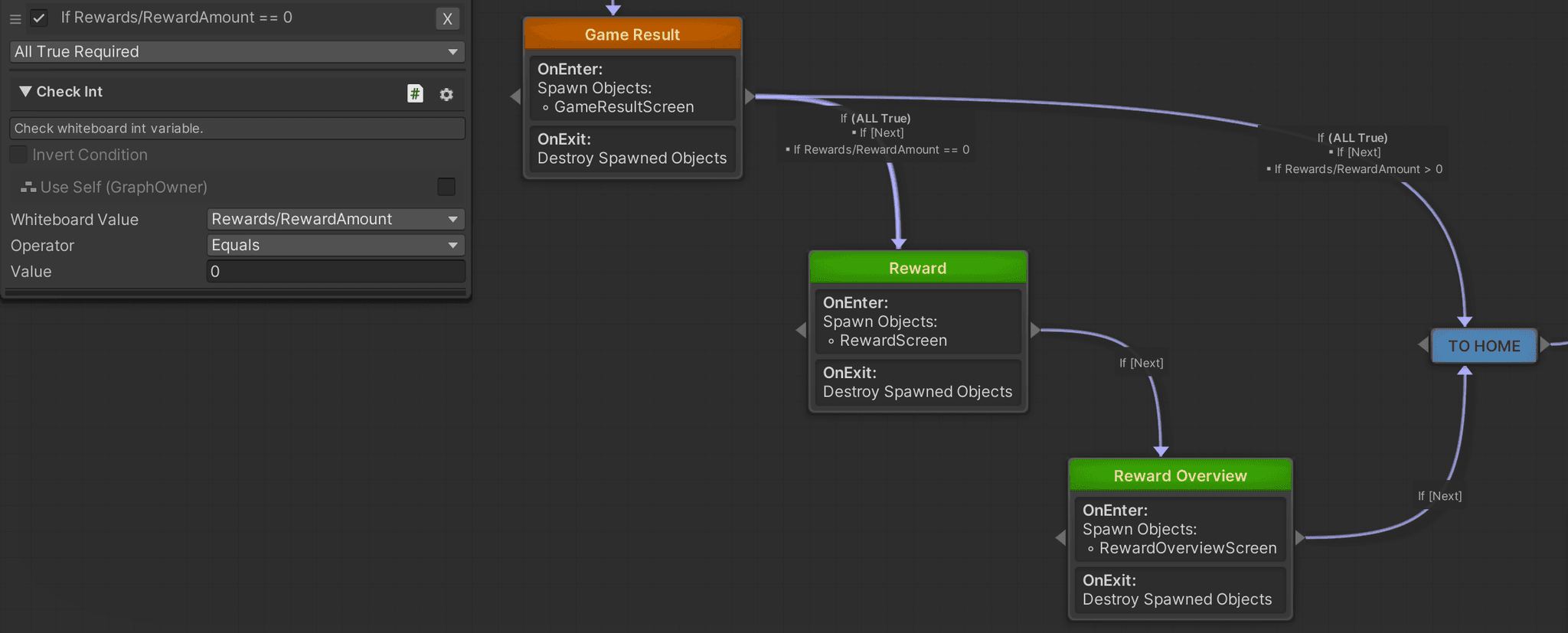
The Check Whiteboard Equals
flow condition allows selecting 2 whiteboard values to see if they are the same.
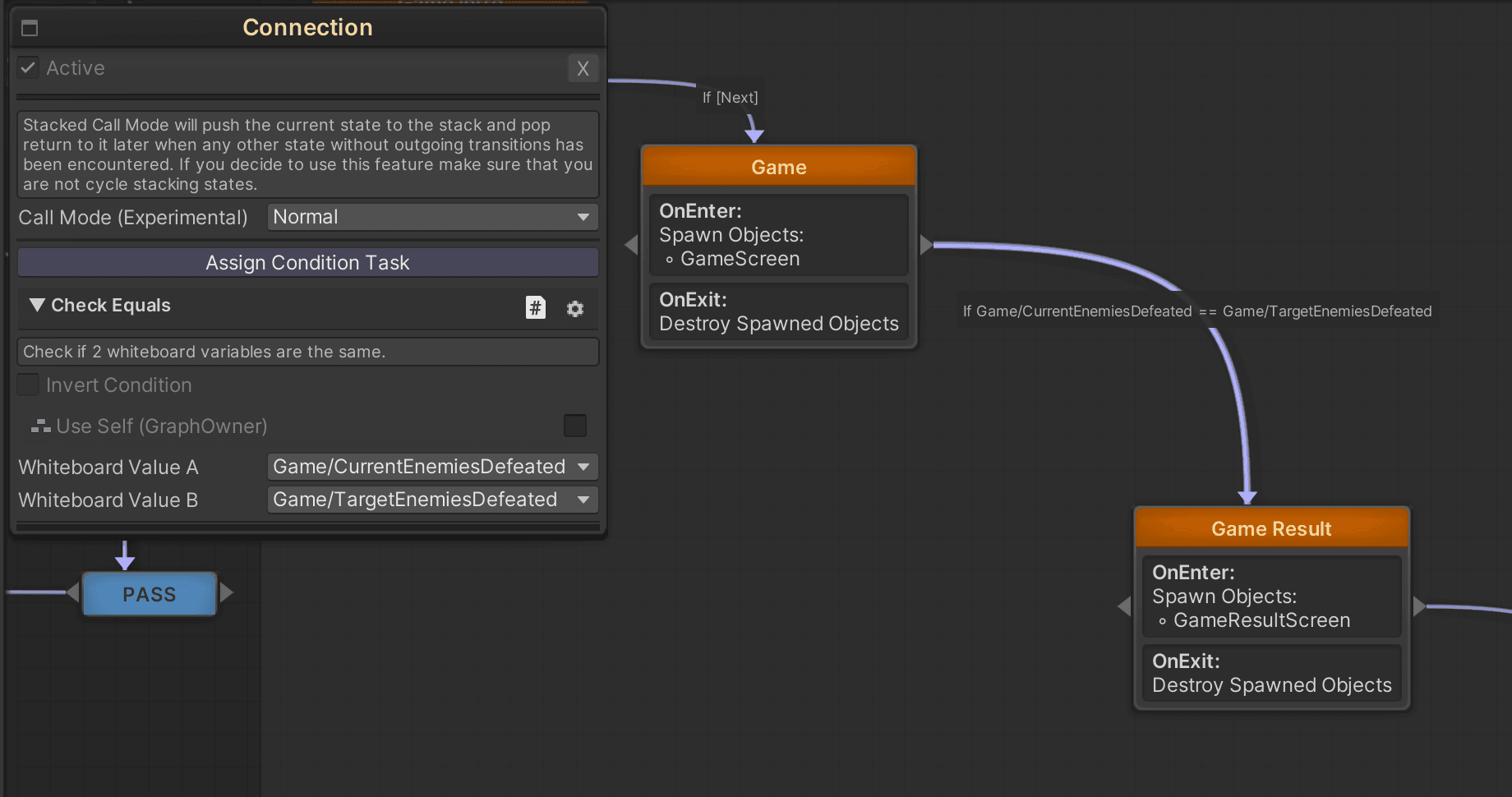
Custom Conditions
Flow conditions can be easily made using the template below. The only mandatory value to implement is OnCheck
. The info
parameter is optional and is the text displayed on the transition line from one node to the next.
namespace Game
{
[Category("Game")]
[Name("ABTest")]
[Description("Check if an AB Test experiment is active or not")]
public class ABTestCondition : ConditionTask<GraphOwner>
{
[FlowLibrary(typeof(ExperimentLibrary))]
public string Experiment;
protected override string info
{
get
{
if (Guid.TryParse(Experiment, out Guid guid) &&
Libraries.Get<ExperimentLibrary>().TryGet(guid, out ExperimentAsset experimentAsset))
{
return "ABTest: " + experimentAsset.Name;
}
return "ABTest: None";
}
}
protected override bool OnCheck()
{
if (Guid.TryParse(Experiment, out Guid guid))
{
return SaveData.Get<ABTestSaveData>().ActiveExperiments.Contains(guid);
}
return false;
}
}
}
As node canvas is not running on Odin Serializer, unfortunately Guids cannot be used, among other inspector values such as interfaces, therefore we use the helper attribute [FlowLibrary(typeof(ExperimentLibrary))]
on a string which we then have to parse to a Guid.