Blackboard
The blackboard allows storing and accessing variables in a specific graph or the entire flow.
This is very useful to remember UI and game states, communicating across different graphs, and making it easier to debug and find issues.
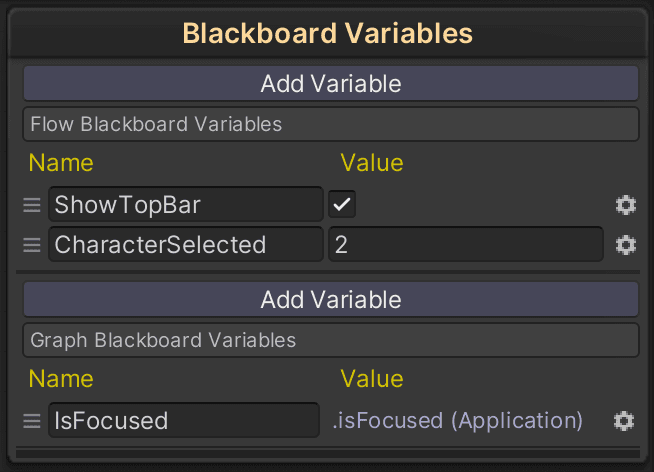
Flow variables can be seen as global variables and graph variables as private variables. Each graph can have its own separate blackboard variables, which can only be reached from within the same graph level. Having multiple instances of the same graph will have its own separate blackboard variables.
Dynamic variables can also be assigned, such as in the example above where the Application is focused. This variable can be used in many different places on node actions and transition conditions.
Defining blackboard variables on the entire flow level, allows access from within any graph in the flow. The flow blackboard variables will appear on the Blackboard component next to the FSM Owner component in the Main scene's Flow GameObject:
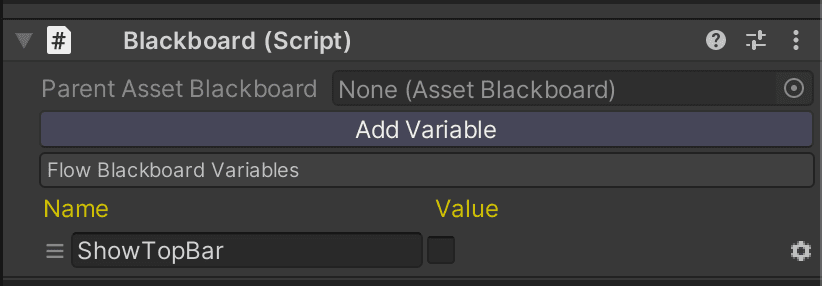
You can utilize blackboard variables from flow conditions as well. In this case we check for a boolean to be true, where we can select the ShowTopBar variable we just created.
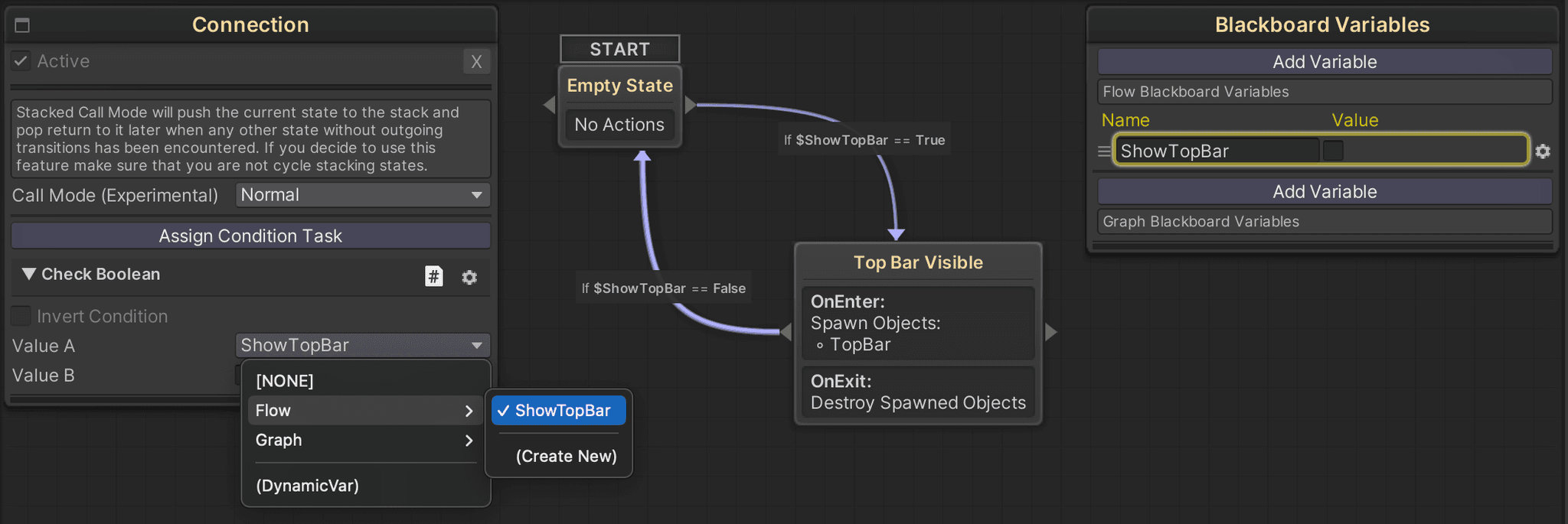
With this flow setup, we can now set the ShowTopBar variable to true or false to show or hide from anywhere in the flow. The next node shows the top bar when entering the home screen, and hides the top bar when exiting:
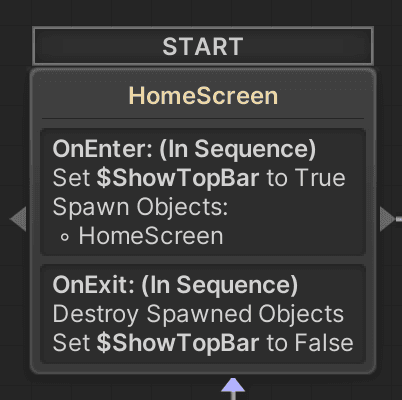
!!! tip "Save and load blackboards" Blackboards can be saved and loaded at any time, allowing for storing the state of a complex dialog tree or the progress through the app, acting as a save data system of sorts. Read the SaveData chapter on how to save a blackboard.
Accessing the blackboard
The global flow blackboard is accessible from anywhere in the code:
protected void Start()
{
Services.Get<FlowService>().SubscribeToBlackboard(BlackboardAssigned);
}
private void BlackboardAssigned(Blackboard blackboard)
{
// Get or set blackboard variables.
}
In case a local blackboard is required, you would need to pass the node which contains the specific flow.
[Node]
public Guid Node;
protected void Start()
{
Services.Get<FlowService>().SubscribeToFlow(Node, FlowAssigned);
}
private void FlowAssigned(List<FSM> flows)
{
// Get or set blackboard variables.
for (int i = 0; i < flows.Count; i++)
{
IBlackboard blackboard = flows[i].blackboard;
}
}
Writing to the blackboard
Writing variables to any blackboard is easy, pass in the name and the value, which can be anything, including LibraryAssets, custom structs or guids.
blackboard.SetVariableValue("variableName", value);
The Set Blackboard Variable
component can also be used to write values to the blackboard from GameObjects, such as setting setting the ShowLoading to true when pressing a button.
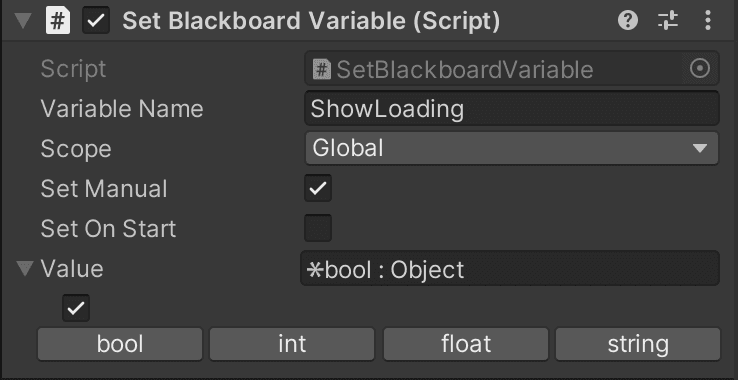
Values do not need to pre-exist
If you need to read and write a value type that does not exist in the blackboard, just leave it out as it does not need to pre-exist at build time. When writing to a blackboard and a value does not exit, it will be created on the fly, supporting any type of data.
Reading from the blackboard
Reading from the blackboard can be done by getting a Variable<T>
.
Variables have a value, but can also be subscribed to for changes or deletion.
Variable<int> variable = blackboard.GetVariable<int>("variableName");
int levelIndex = variable.value;
variable.onValueChanged += OnValueChanged;
private void OnValueChanged(object value)
{
int newLevelIndex = (int)value;
}
Blackboard events
The real power of blackboards comes in to play when using the Blackboard Event component. It allows to listen to any changes and either check if they have changed or are a specific value. When valid, it will execute a unity event.
This is an easy way to communicate between objects, such as in this example where the loading screen canvas is enabled and disabled by listening to the ShowLoading
blackboard variable changes.
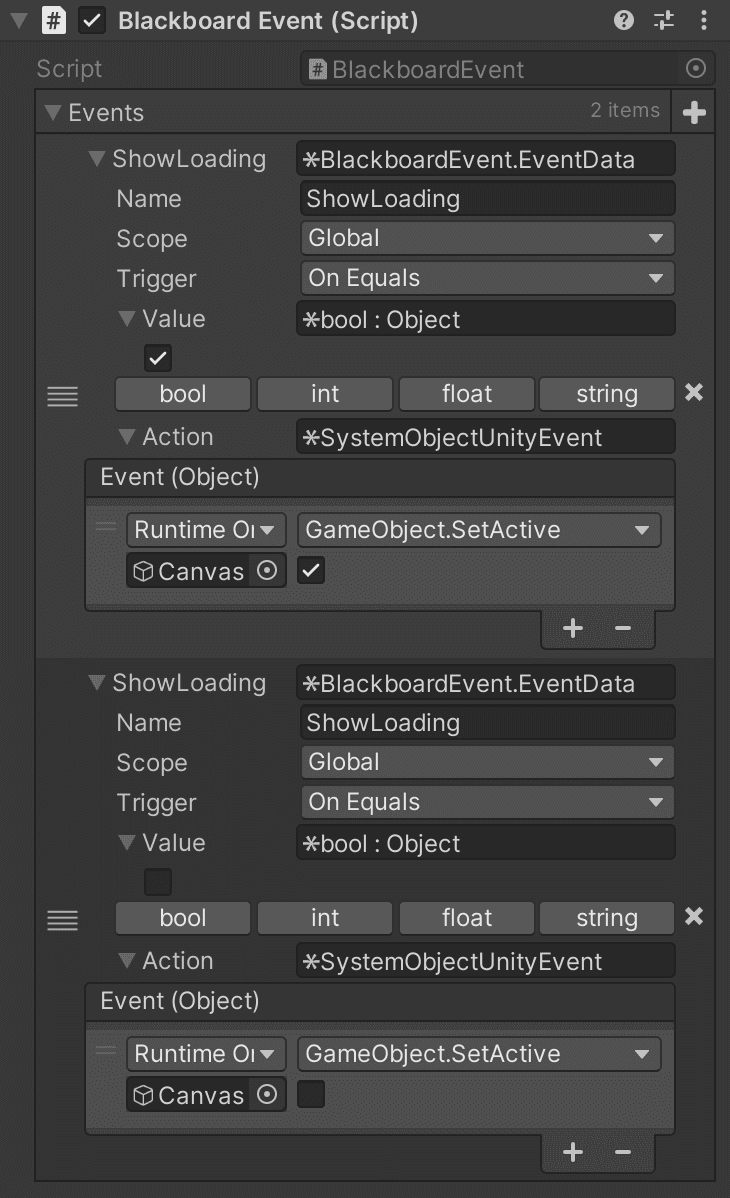